Exploring Koa.js with Deno: Building a Modern Backend
In the evolving world of web development, the quest for more efficient and scalable backend solutions is perpetual. With the advent of Deno, a modern JavaScript/TypeScript runtime, and Koa.js, a flexible Node.js web framework, building server-side applications has taken an intriguing turn.
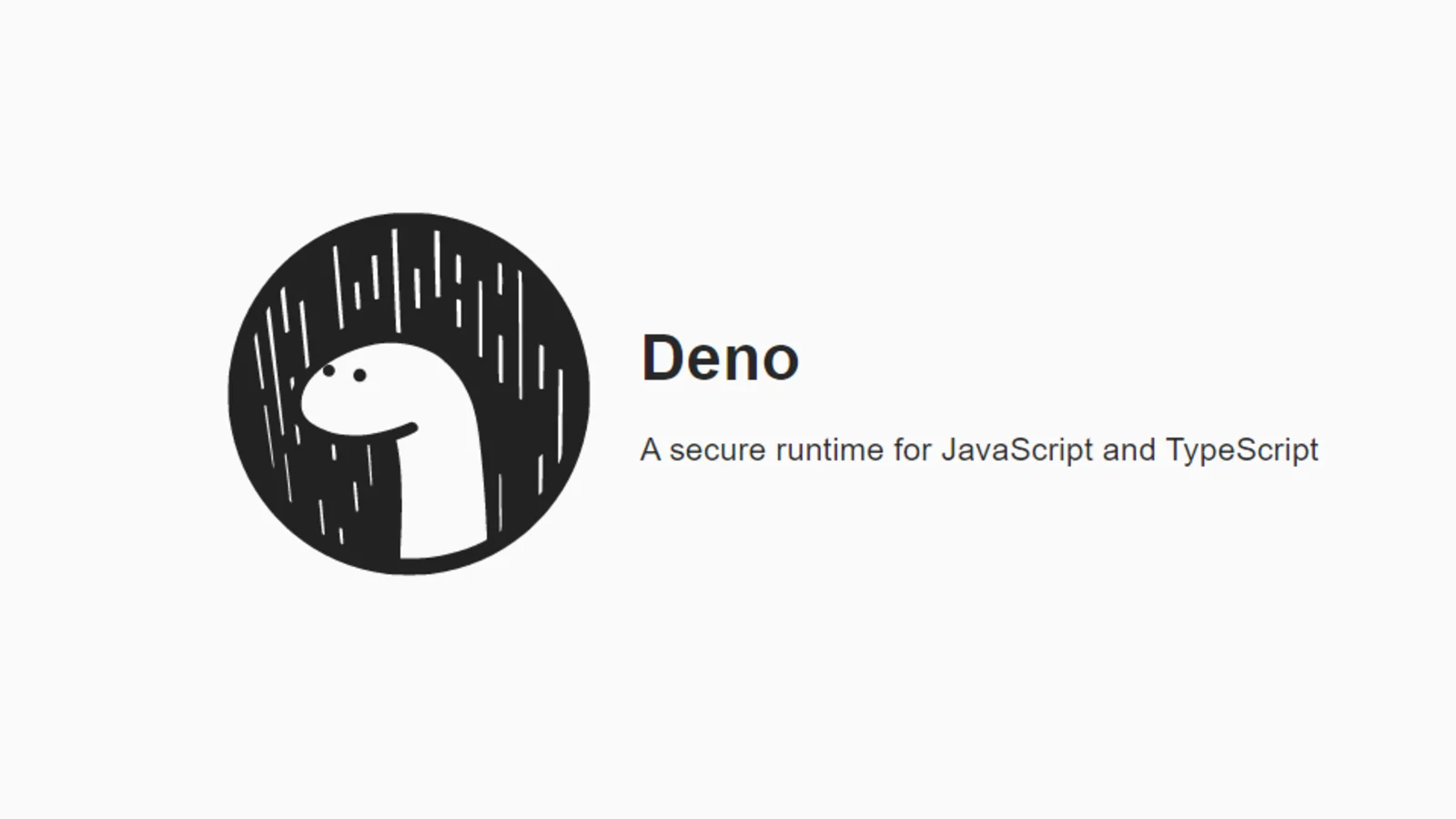
Introduction to Deno
Deno, created by Ryan Dahl, the original creator of Node.js, addresses many of Node.js's shortcomings while introducing several modern features:
- Secure by default
- Supports TypeScript out of the box
- Ships with a single executable
Deno's approach to module management and its emphasis on security make it an excellent choice for building web applications.
Why Koa.js with Deno?
Koa.js, known for its minimalistic approach and flexibility, offers a great way to build web applications and APIs. When combined with Deno, Koa.js benefits from Deno's modern features, such as top-level await and native TypeScript support.
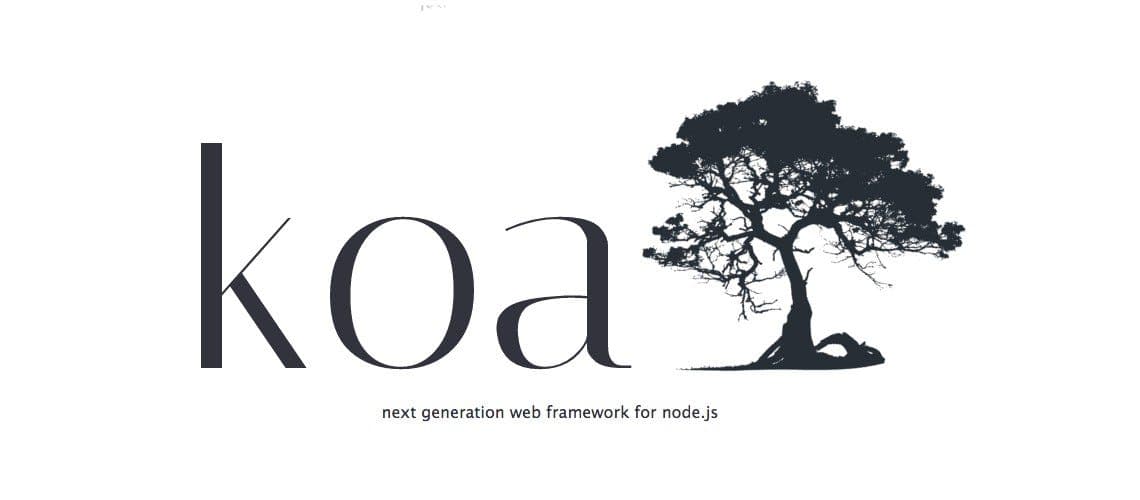
Setting Up a Koa.js Server in Deno
To get started with Koa.js in Deno, you first need to set up your Deno environment. Here's a simple guide:
-
Install Deno: Follow the instructions on the Deno installation page.
-
Create Your Koa.js Server: Write your Koa.js server script using Deno's module imports.
-
Run Your Server: Use the
deno run
command with the necessary permissions.
Example Code
import Koa from 'https://deno.land/x/koa/mod.ts';
const app = new Koa();
app.use((ctx) => {
ctx.body = 'Hello from Koa in Deno!';
});
app.listen(3000);
Integrating Middleware
Koa's real power lies in its middleware framework. Here's how you can integrate custom middleware for logging, error handling, and more.
app.use(async (ctx, next) => {
try {
await next();
} catch (err) {
ctx.status = err.status || 500;
ctx.body = err.message;
ctx.app.emit('error', err, ctx);
}
});
A short video example about Deno
Conclusion
Combining Koa.js with Deno offers a modern approach to building backend applications. The simplicity of Koa.js and the robust features of Deno create a powerful duo for developers looking to build efficient and secure web applications.